Android SDK
Overview
Section titled “Overview”The Secure Privacy Consent Management SDK provides developers with an easy way to manage user consent within their applications. By integrating this SDK, you can ensure compliance with privacy regulations while offering users control over their data preferences.
Getting Started
Section titled “Getting Started”Obtain Your Application ID
Section titled “Obtain Your Application ID”To use the Secure Privacy Consent Management SDK, you need an Application ID. Sign up for a free trial at Secure Privacy to get your Application ID.
Installation
Section titled “Installation”To begin using the Secure Privacy SDK for Android, include the SDK dependency in your project along with some additional dependencies.
- Add the SDK dependency to your
build.gradle
:
dependencies {
implementation("ai.secureprivacy.sdk:mobileConsent:0.2.4-alpha")
// Standard libraries implementation(libs.androidx.core.ktx) implementation(libs.androidx.appcompat) implementation(libs.material)
// Required libraries for the SDK implementation(libs.retrofit) implementation(libs.gson) implementation(libs.converterGson) implementation(libs.converterScalars) implementation(libs.cryptoKtx)}
- Ensure the following library versions are included in your
libs
configuration:
retrofit = "2.11.0"gson = "2.11.0"converterGson = "2.11.0"converterScalars = "2.11.0"cryptoKtx = "1.1.0-alpha06"
Initialization
Section titled “Initialization”To initialize the SDK, call the following method with your applicationId
in your main activity or app initialization code. You may also include secondaryApplicationId
, which is an optional parameter used to display a secondary consent banner in your app:
val spConsentEngine = SPConsentEngine.initialise( activity, SPAuthKey( applicationId = Config.APPLICATION_ID, secondaryApplicationId = Config.SECONDARY_APPLICATION_ID ))
This will set up the SDK and enable access to its features and returns an instance of SPConsentEngine
.
Consent Status
Section titled “Consent Status”You can check the current consent status by calling:
spConsentEngine.getConsentStatus(Config.APPLICATION_ID)
Consent States
Section titled “Consent States”The SDK supports three consent states:
- Collected: Consent has already been obtained.
- Pending: Consent needs to be collected.
- UpdateRequired: Consent needs to be re-collected.
Check if User has Enabled the Package
Section titled “Check if User has Enabled the Package”To check if a specific package has been marked as enabled by the user upon giving consent, you can call:
val package = spConsentEngine?.getPackage(packageId, Config.APPLICATION_ID)?.dataif(package!=null && package.isEnabled){ //enable features}
This would return a SPDataMessage containing the SPMobilePackage only if the user has consented. Otherwise, it would return null.
Collecting Consent
Section titled “Collecting Consent”To launch the consent collection popup, use the following method:
ConsentBanner.show(activity);
This popup will inform users about the SDK and provide them with options to agree to all or deny consent.
Additionally, you can call the following method to display the secondary consent banner:
ConsentBanner.showSecondary(activity);
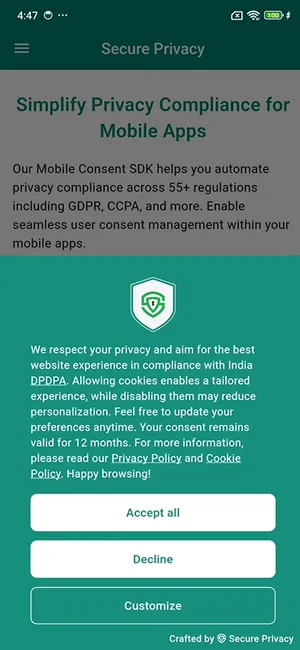
Listening to Consent Events
Section titled “Listening to Consent Events”You can listen to consent events in your activity by adding and removing listeners in the onStart
and onStop
methods:
override fun onStart() { super.onStart() SPConsentEngine.addListener( MOBILE_CONSENT_EVENT_CODE, object : SPConsentEventListener { override fun onConsentAction(data: SPDataMessage<SPConsentEvent>) { //Custom logic } } )}
override fun onStop() { SPConsentEngine.removeListener(YOUR_UNIQUE_EVENT_CODE) super.onStop()}
Here, MOBILE_CONSENT_EVENT_CODE
is a unique code you can use to register/unregister events and track updates on consent actions.
Important Note: The response from the onConsentAction
callback may come from a background thread. Be sure to handle thread switching (e.g., using Handler
, runOnUiThread()
, or lifecycleScope
) when updating the UI, as UI updates must occur on the main thread.
Additional Way to Listen to Consent Events:
As an additional way to listen to consent events, you can now observe consent events using the following method:
SPConsentEngine.getConsentEventsData(YOUR_UNIQUE_EVENT_CODE) .observe(activity) { data -> //Custom logic }
Event Codes
When using the SDK to listen to consent events, it’s important to understand how event codes work.
-
Event Codes: Event codes are unique identifiers that is used to register/unregister consent events. The event code helps distinguish between different consent events, particularly when dealing with multiple listeners or consent events across different activities or classes.
-
Automatic Event Code Registration: When you call
getConsentEventsData(UNIQUE_EVENT_CODE)
with an event code, the SDK automatically registers that event code. You do not need to manually register the event code, as this is handled internally by the SDK. This ensures that the event codes are uniquely associated.
Customization Options
Section titled “Customization Options”The consent banner supports customization options and displays a “Customize” button that allows users to adjust their consent preferences. When they click the customization button, they will be directed to the Preference Center, where they can:
- View a list of frameworks and their services.
- Enable or disable consent for specific services.
To display the Preference Center directly, simply start the Preference Center activity:
SPPreferenceCenter.show(context, applicationId)
Declaring SPPreferenceCenter activity in the Manifest
Section titled “Declaring SPPreferenceCenter activity in the Manifest”To properly use the Preference Center Activity, you need to declare it in your app’s AndroidManifest.xml
file. Add the following line inside the <application>
tag:
<activity android:name="ai.secureprivacy.mobileconsent.ui.preference_center.SPPreferenceCenter" android:theme="@style/Theme.SecurePrivacyMobile"/>
This ensures that the SDK’s Preference Center activity is registered and can be accessed correctly.
Clearing Session
Section titled “Clearing Session”To clear the session, call clearSession()
. This method is useful when you want to ensure that all local session-specific sdk data is cleared. It is typically used when a user logs out or resets their preferences.
spConsentEngine.clearSession()
Handling Unique Client ID (optional)
Section titled “Handling Unique Client ID (optional)”Each time the app is installed (fresh install) or during a new session, Secure Privacy Mobile Consent SDK generates a unique clientId for the user. This clientId can be retrieved by calling the following method.
val clientId = spConsentEngine.getClientId(Config.APPLICATION_ID)
This clientId
can be used to associate the user’s consent with internal identifiers within your app or backend systems. You can also use this clientId for custom user identification and to ensure accurate tracking of consent preferences.
Essential Services
Section titled “Essential Services”You can mark certain services as essential. These services will not be optional for the user to deny, ensuring compliance with the app’s required functionalities.
Privacy and Cookie Policy
Section titled “Privacy and Cookie Policy”The Preferences screen also includes tabs showcasing content related to the app’s privacy and cookie policies. This ensures users have access to essential information regarding their data handling.
Data Requests
Section titled “Data Requests”Users can submit a request for their data by filling out a form in the Request Data section. This feature helps manage user data requests in compliance with privacy regulations.
Explore the Example App
Section titled “Explore the Example App”Check out Secure Privacy Android SDK – Example App on GitHub!